Database Insert examples
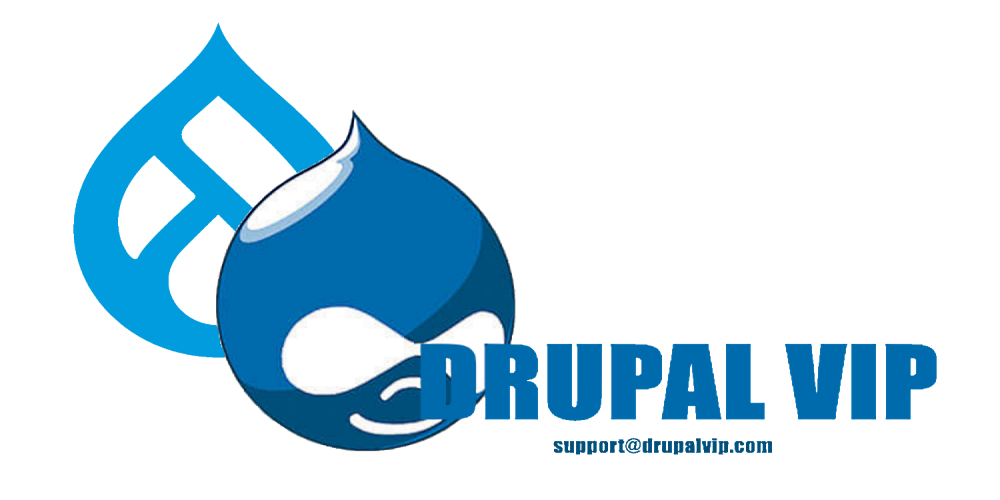
An insert query adds new data to a database table.
Just before you try the example make sure you know the desired table and its columns.
This is how you do it Drupal 10
Insert queries must always use a query builder object.
Certain databases require special handling for LOB (Large OBject, such as TEXT in MySQL) and BLOB (Binary Large OBject) fields, so a layer of abstraction is required to allow individual database drivers to implement whatever special handling they require.
The insert query object uses a fluent API.
That is, all methods (except execute()) return the query object itself allowing method calls to be chained. In many cases, that means the query object will not need to be saved to a variable at all.
The insert query object supports a number of different usage patterns to support different needs.
In general, the workflow consists of specifying the fields that the query will insert into, specifying the values the query will insert for those fields, and executing the query.
$query = \Drupal::database()->insert('ttn_mysql_table');
$query->fields([ 'name' => 'Rajveer', 'age' => 10,]);
$query->execute();
$connection = \Drupal::service('database');
$result = $connection->insert('mytable')
->fields([
'title' => 'Example',
'uid' => 1,
'created' => \Drupal::time()->getRequestTime(),
])
->execute();
Multi-Insert
$values = [
['title'=>'Example','uid'=>1,'created'=>\Drupal::time()->getRequestTime() ],
['title'=>'Example 2','uid'=>1,'created'=>\Drupal::time()->getRequestTime() ],
['title'=>'Example 3','uid'=>2,'created'=>\Drupal::time()->getRequestTime() ],
];
$query = $connection->insert('mytable')->fields(['title', 'uid', 'created']);
foreach ($values as $record) {
$query->values($record);
}
$query->execute();
Insert based on Select results
// Build the SELECT query.
$query = $connection->select('node_field_data', 'n');
// Join to the users table.
$query->join('users', 'u', 'n.uid = u.uid');
// Add the fields we want.
$query->addField('n','nid');
$query->addField('u','name');
// Add a condition to only get page nodes.
$query->condition('type', 'page');
// Perform the insert.
$connection->insert('mytable')
->from($query)
->execute();
insert() OR query()
This is a commonly asked question. (See the comments on this page.) What are the difference between insert() and query()?
insert() has each column specified as a separate entry in the fields array and the code can clean each column value. query() has an SQL string with no way of checking individual columns. If you use query() with placeholders, the code can check the column values but placeholders are just an option, there is no way to ensure your SQL does not contain values not passed through placeholders.
insert() passes the request through a set of hooks to let other modules check and modify your requests. This is the right way to work with other modules. query() is slightly faster because query() does not pass the request through the hooks. You might save processing time but your code will not let other modules help your code.
insert() is more likely to work with other databases and future versions of Drupal.
function deprecated: db_insert
function was deprecated in drupal:8.0.0 and last version that support it was D8.9,
it was removed from drupal:9.0.0.
Instead, get a database connection injected into your service from the container and call insert() on it.
For example, $injected_database->insert($table, $options);