Fetching data using inbuilt fetch API
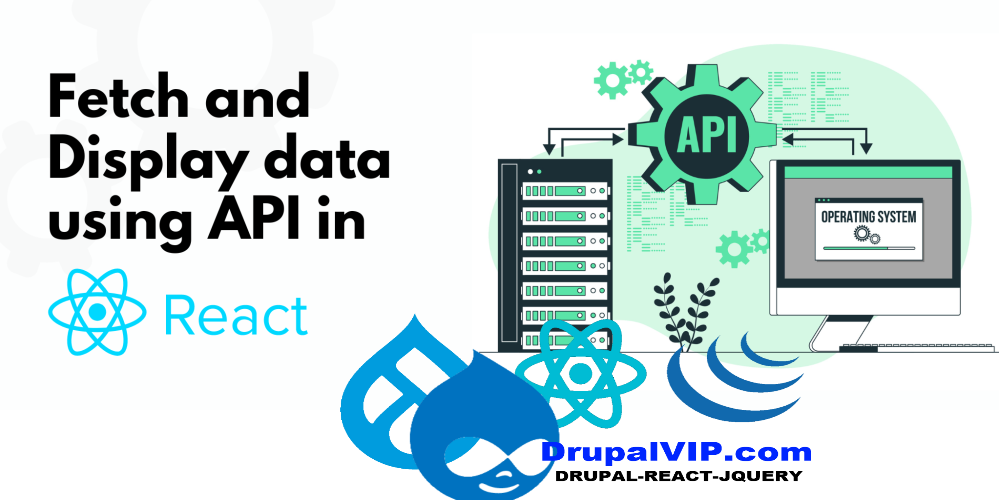
import React, { useEffect, useState } from "react"
const UsingFetch = () => {
const [users, setUsers] = useState([])
const fetchData = () => {
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => {
return response.json()
})
.then(data => {
setUsers(data)
})
}
useEffect(() => {
fetchData()
}, [])
return (
<div>
{users.length > 0 && (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)}
</div>
)
}
export default UsingFetch
In the above code,
- We have are using a useEffect hook, which will be executed once the component is mounted (alternative of componentDidMount in class-based components).
- Inside the useEffect hook, we are calling fetchData function.
- In the fetchData function, we are making the API call to fetch users and set the users to a local state.
- If users exist, then we are looping through them and displaying their names as a list.
Since the API calls are asynchronous, fetch API returns a Promise.
Hence, we chain the then method with a callback, which will be called when we receive the response from the server/backend.
Since we need the response to be resolved to a JSON, we call .json() method with the returned response.
Again .json() return a promise, therefore we need to chain another then method to resolve the second promise.
Since the then callbacks have only one line, we can use implicit returns to shorten the fetchData method as follows:
const fetchData = () =>
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => response.json())
.then(data => setUsers(data))
note:
The Data loop, if creating an element in a render method, make sure it has a 'key' property
<li key={user.id}>{user.name}</li>
Code Snippet
import React, { useEffect, useState } from "react"
const UsingFetch = () => {
const [users, setUsers] = useState([])
const fetchData = () => {
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => {
return response.json()
})
.then(data => {
setUsers(data)
})
}
useEffect(() => {
fetchData()
}, [])
return (
<div>
{users.length > 0 && (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)}
</div>
)
}
export default UsingFetch