How to get the current request object
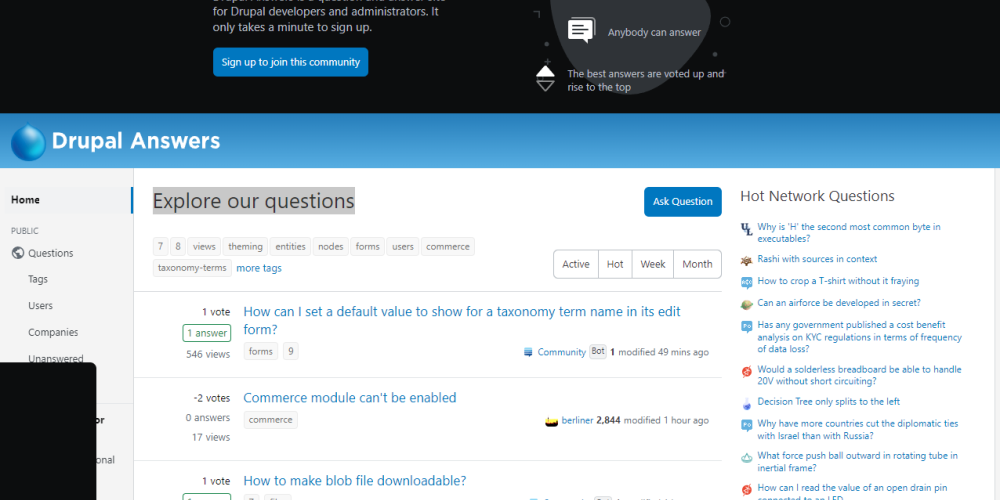
Procedural
In procedural code get the request from the static wrapper \Drupal
:
$request = \Drupal::request();
Service
In a service get the current request from the injected argument @request_stack
:
The module_name/module_name.services.yml
file :
services:
custom.service:
class: Drupal\module_name\Service\CustomService
arguments:
- '@request_stack'
The module_name/src/Service/CustomService.php
file :
use Symfony\Component\HttpFoundation\RequestStack;
/** @var \Symfony\Component\HttpFoundation\RequestStack */
protected $requestStack;
class customService
{
public function __construct(RequestStack $requestStack) {
$this->requestStack = $requestStack;
}
public function doSomething() {
// use $this->requestStack->getCurrentRequest()
}
}
Controller
In a controller you can pull the request from the route argument stack by including a typed request parameter. An example from UserController
:
use Symfony\Component\HttpFoundation\Request;
public function resetPass(Request $request, $uid, $timestamp, $hash) {
// use $request
}
It's not necessary to define the route parameter in the route definition. The request is always available.
Form
In a form method get the request from getRequest() :
public function submitForm(array &$form, FormStateInterface $form_state) {
$request = $this->getRequest();
...
}
Don't use the injected requestStack
property directly, because it is not available for all Form API callbacks.
The service needs @request_stack
as argument.
You can find examples in core.services.yml.
if injected directly it would be available, but you can't be sure it is when using the form class in Form API.
$request = $this->getRequest()
gets the request from the injected service, but has a fallback in case it is not available.
public static function Drupal::request
Retrieves the currently active request object.
Note: The use of this wrapper in particular is especially discouraged.
Most code should not need to access the request directly.
Doing so means it will only function when handling an HTTP request, and will require special modification or wrapping when run from a command line tool, from certain queue processors, or from automated tests.
If code must access the request, it is considerably better to register an object with the Service Container and give it a setRequest()
method that is configured to run when the service is created.
That way, the correct request object can always be provided by the container and the service can still be unit tested.
If this method must be used, never save the request object that is returned.
Doing so may lead to inconsistencies as the request object is volatile and may change at various times, such as during a subrequest.