ResourceResponse When and How
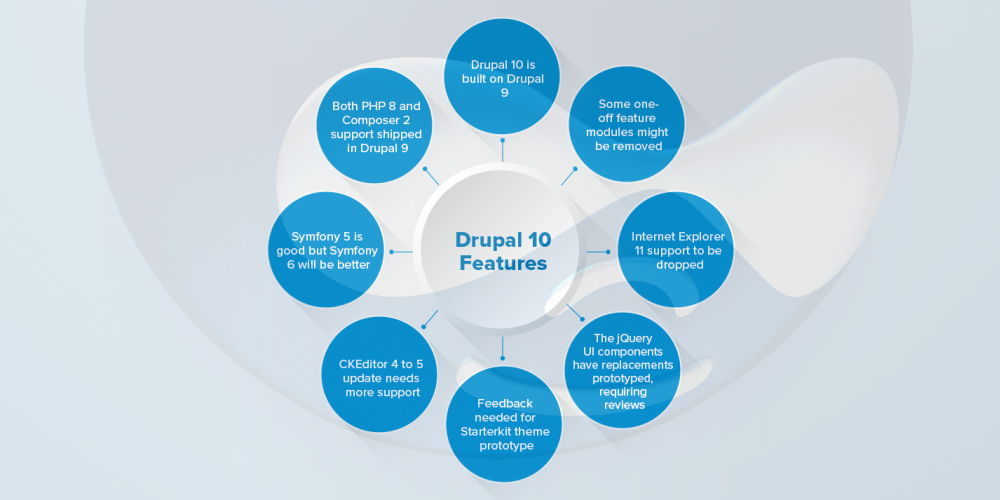
Creating the Resource Plugins
In order to create a custom REST service, you will need to become acquainted with Plugins.
Plugins are small pieces of functionality that are swappable.
Plugins that perform similar functionality are of the same plugin type.
In the case of our custom resource, we need to extend the ResourceBase plugin, which can be found here
In order to implement a ResourceBase plugin in our REST module, create a new fileDemoResource.php in /src/Plugin/rest/resource/ directory of the Demo REST API module and add the following:
namespace Drupal\demo_rest_api\Plugin\rest\resource;
use Drupal\rest\Plugin\ResourceBase;
use Drupal\rest\ResourceResponse;
class ExampleHello extends ResourceBase {
/**
* {@inheritdoc}
*/
public static function create(/* ... */) {
return new static(/* ... */);
}
/**
* Constructs a Drupal\rest\Plugin\ResourceBase object.
*/
public function __construct(/* ... */) {
parent::__construct(/* ... */);
// ...
}
/*
* Responds to GET requests.
*/
public function get() {
$account = \Drupal::currentUser()->getAccount();
if (!$account->id()) {
return new ResourceResponse(array(
'welcome' => 'visitor'
));
}
return new ResourceResponse(array(
'hello' => $account->getAccountName()
));
}
}
class ModifiedResourceResponse
A response that does not contain cacheability metadata.
Used when resources are modified by a request: responses to unsafe requests (POST/PATCH/DELETE) can never be cached.
In order to user library Drupal\rest
, you must activate the module : RESTful Web Services
Code Snippet
$response = ['message' => 'Hello, this is a rest service'];
return new ResourceResponse($response);
use Symfony\Component\HttpFoundation\Response;
$response = new Response(
'Content',
Response::HTTP_OK,
['content-type' => 'text/html']
);
$response->setContent('Hello World');
// the headers public attribute is a ResponseHeaderBag
$response->headers->set('Content-Type', 'text/plain');
$response->setStatusCode(Response::HTTP_NOT_FOUND);