custom RESTful API endpoint in Drupal 7
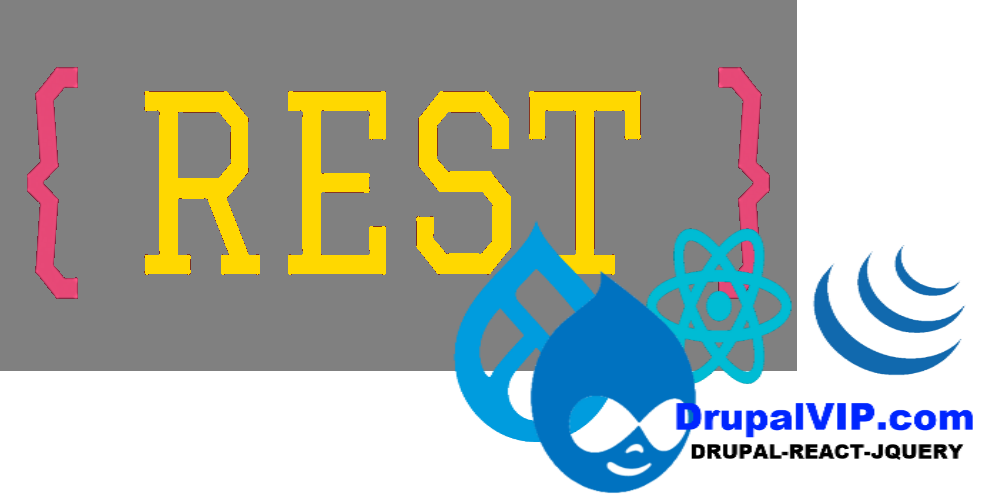
RESTful API endpoints in Drupal can allow you to expose the content of Drupal 7 website to other external resources.
These endpoints can be used for fetching data with an AJAX call or simply a web service.
For making complex RESTful web services in Drupal 7, Services module is the best option to go for but if you looking for a simple endpoint in Drupal 7, which outputs JSON response, then going with a custom solution will be the best bet.
About the Service module:
For newer versions of Drupal, we recommend to use the Drupal core JSON:API or RESTful Web services modules.
A standardized solution for building API's so that external clients can communicate with Drupal.
Out of the box it aims to support anything Drupal Core supports and provides a code level API for other modules to expose their features and functionality via HTTP.
It provide Drupal plugins that allow others to create their own authentication mechanisms, request formats, and response formats.
Creating simple endpoint in Drupal 7
Let's start by updating our module main file: my_module.module, with menu hook support
/**
* Implements hook_menu().
*/
function mymodule_menu() {
$items['api/mymodule'] = array(
'access callback' => true,
'page callback' => 'mymodule_json',
'delivery callback' => 'drupal_json_output',
);
return $items;
}
The hook menu is defining an application endpoint, the above endpoint is being defined with callback function and output type, in our case JSON type
A noticeable point in this callback is the "delivery callback" argument which runs the output through drupal_json_output function.
/**
* Callback function to return the data.
*/
function mymodule_json() {
$data = ['cat', 'dog', 'mouse', 'elephant'];
return $data;
}
if you navigate to the callback url 'api/mymodule' to see the output then you will see the following result
[
"cat",
"dog",
"mouse",
"elephant",
]
This output is exposed as a JSON and can be consumed by other platforms or an AJAX callback to consume data.
The same approach can be used to expose a node or a user as a JSON output.